React, also known as ReactJS, is a widely used JavaScript library for building user interfaces, particularly for single-page applications. Developed by Facebook, it has revolutionized the way we create dynamic and interactive web applications. This guide aims to provide an in-depth understanding of how to learn React JS in 2024, its key concepts, features, and practical applications. Whether you’re a beginner or looking to deepen your knowledge, this article covers everything you need to know about React.
What is React?
React is a JavaScript library specifically designed for building user interfaces (UIs) on the web. It follows a declarative, component-based approach, allowing developers to create reusable UI components. React is known for its use of the Virtual DOM, which optimizes rendering performance by minimizing direct manipulations of the actual DOM. This makes React not only fast but also highly compatible with other tools and libraries.
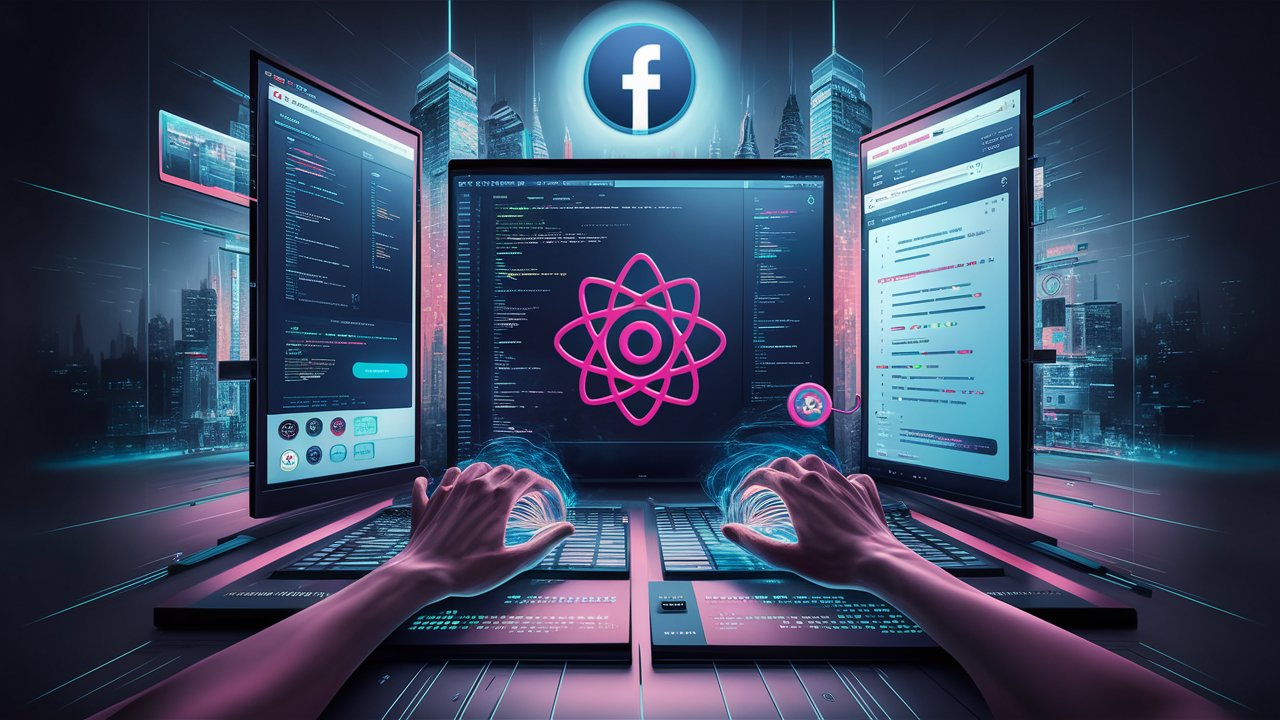
Prerequisites for Learning React
Before diving into React, it’s essential to have a basic understanding of several key technologies:
- HTML and CSS: Essential for structuring and styling web pages.
- JavaScript and ES6: JavaScript is the core language of React, and understanding modern JavaScript (ES6) features is crucial.
- JSX (JavaScript XML) & Babel: JSX is a syntax extension for JavaScript that looks similar to HTML. Babel is a tool that transpires JSX into standard JavaScript.
- Node.js and npm: Used for managing dependencies and running React applications.
- Git and Command Line Interface (CLI): Useful for version control and executing commands.
History of React
React was created by Facebook developers who sought a more efficient way to handle complex UIs. The traditional DOM was found to be slow, leading to the development of the Virtual DOM. React’s first release was in 2013, and it quickly gained popularity due to its performance benefits and developer-friendly features. The current stable version is React 18.2.0, released on June 14, 2022. The library continues to evolve, incorporating new features with each update.
How React Works
React uses a Virtual DOM, which is a lightweight representation of the actual DOM. When a component’s state or props change, React updates the Virtual DOM first, calculates the differences, and then updates the real DOM with minimal changes. This process, known as reconciliation, makes React applications highly efficient, as only the necessary parts of the DOM are updated.
Key Features of React
React offers a robust set of features that make it a preferred choice for developers:
- Component-Based Architecture: React allows breaking down the UI into smaller, self-contained components. Each component can have its own state and props, making it easier to manage and reuse code.
- JSX (JavaScript Syntax Extension): JSX enables developers to write HTML-like syntax in JavaScript files. It enhances readability and expressiveness, making it easier to visualize the structure of components.
- Virtual DOM: The Virtual DOM provides a fast and efficient way to update the UI. React calculates the minimal set of changes required and updates only the affected parts of the real DOM.
- One-way Data Binding: React uses a unidirectional data flow, meaning data flows from parent components to child components. This simplifies the data flow and makes debugging easier.
- Performance Optimization: By using the Virtual DOM and efficient diffing algorithms, React ensures high performance and fast rendering.
- Single-Page Applications (SPAs): React is ideal for building SPAs, where the page dynamically updates without reloading, providing a smoother user experience.
ReactJS Lifecycle
Understanding the lifecycle of React components is crucial for managing state, performing side effects, and optimizing components. The lifecycle consists of three main phases:
- Initialization: The component is constructed with default props and initial state. This usually happens in the constructor method.
- Mounting:
- Constructor: Initializes the component’s state and binds event handlers.
- render(): Returns the JSX representation of the component.
- componentDidMount(): Called after the component is inserted into the DOM, ideal for data fetching and setting timers.
- Updating:
- shouldComponentUpdate(): Determines if the component should re-render. Can be used to optimize performance.
- render(): Called to reflect changes in state or props.
- componentDidUpdate(prevProps, prevState): Called after the component updates, useful for handling side effects.
- Unmounting:
- componentWillUnmount(): Invoked just before the component is removed from the DOM, used for cleanup activities.
Setting Up a React Project
To set up a React project, follow these steps:
- Install Node.js and npm: Download from the Node.js official website.
- Create a new React app:
npx create-react-app my-app
cd my-app
npm start
- Explore the project structure: Familiarize yourself with the files and directories created by
create-react-app
. - Start coding: Modify
src/App.js
to create your first React component.
Practical Examples and Best Practices
Example 1: Creating a Simple Component
import React from 'react';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
export default Greeting;
Example 2: Using State and Props
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
export default Counter;
Best Practices
- Keep components small and focused. Each component should do one thing well.
- Use functional components and hooks instead of class components for simpler code.
- Manage state effectively, using tools like the Context API or Redux when necessary.
- Optimize performance by using techniques like memoization and lazy loading.
- Follow a consistent style and structure for your components.
References and Further Reading
- React Official Documentation
- JavaScript Info – The Modern JavaScript Tutorial
- Learn JavaScript with freeCodeCamp
- CSS-Tricks: A Complete Guide to Flexbox
Conclusion
React is a versatile and powerful library for building modern web applications. Its component-based architecture, efficient Virtual DOM, and support for single-page applications make it an excellent choice for developers. By mastering the fundamentals and exploring advanced topics, you can leverage React to create high-performance, scalable applications. Continue learning and experimenting with React to unlock its full potential. Happy coding!
FAQs on React
Is React a Framework or Library?
React is a library, not a framework. While frameworks provide a full-fledged solution, including structure and rules, React focuses solely on the UI layer. This flexibility allows developers to integrate React with other tools and libraries as needed.
How Do I Start Learning React?
To start learning React, follow these steps:
- Learn the basics of JavaScript, HTML, and CSS.
- Understand React fundamentals, including components, JSX, props, and state.
- Explore advanced topics like hooks, context API, and state management with Redux.
- Build projects to apply your knowledge in real-world scenarios.
For a structured learning path, refer to the React Tutorial.
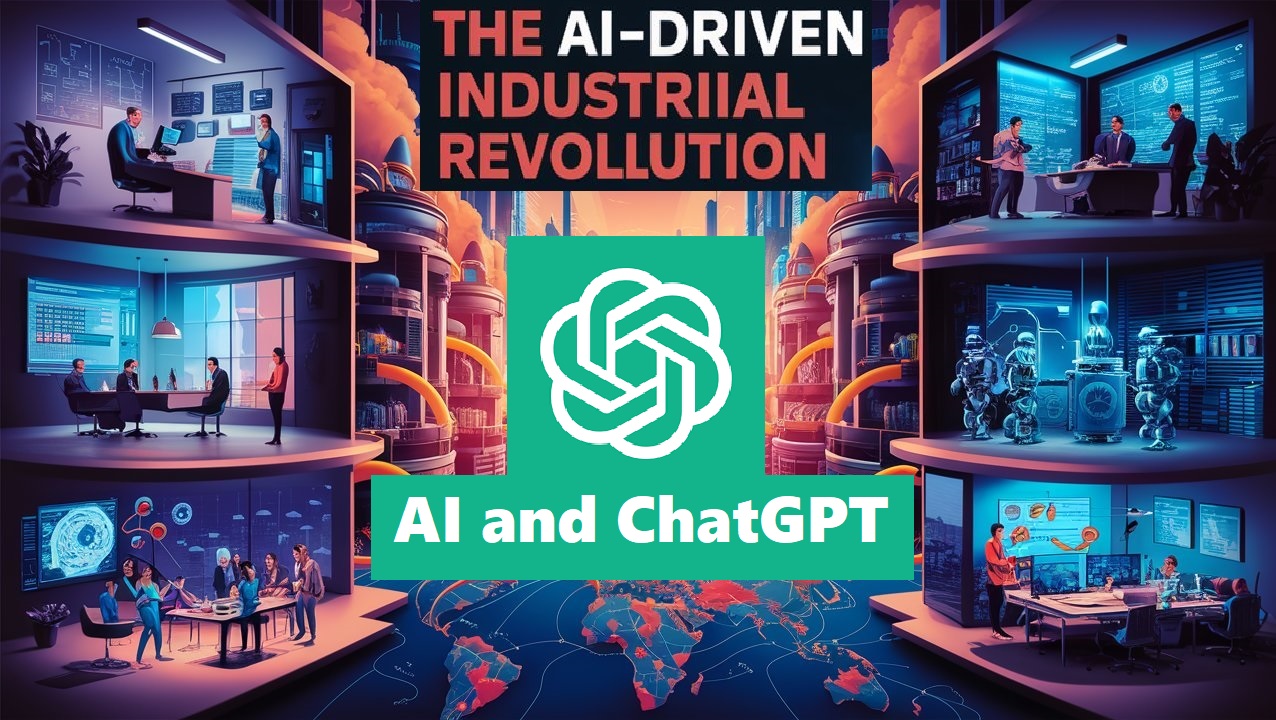
How AI and ChatGPT Technology Transform the Next Industrial Revolution
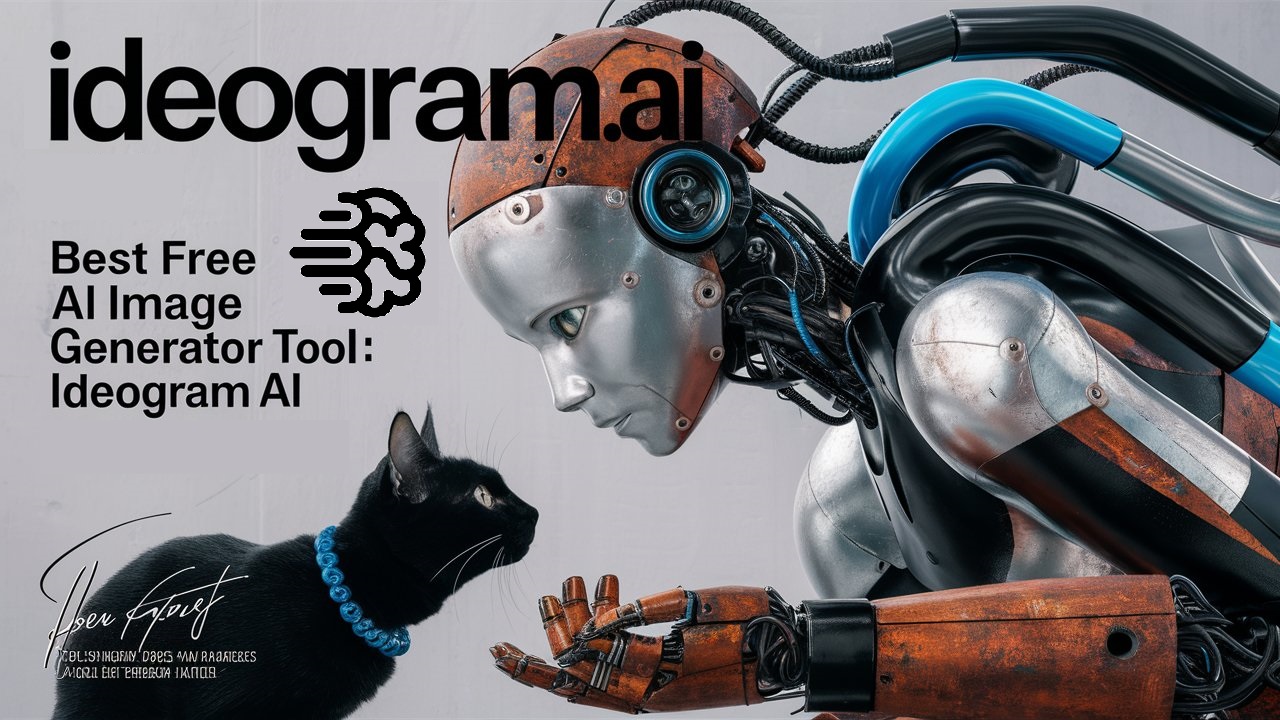
Best Free AI Image Generator Tool: Ideogram AI
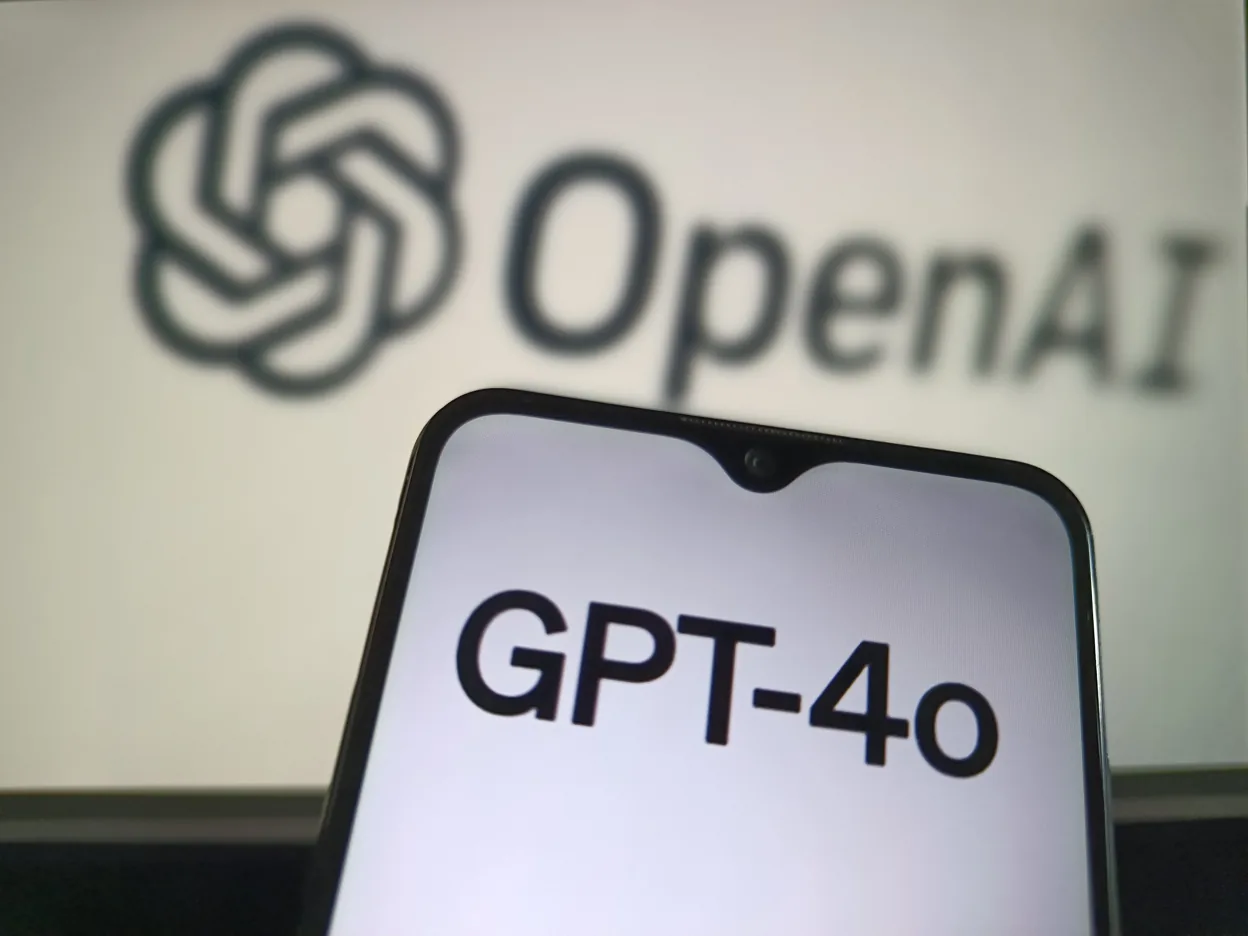
GPT-4o: How ChatGPT-Integrates Audio, Vision, and Text
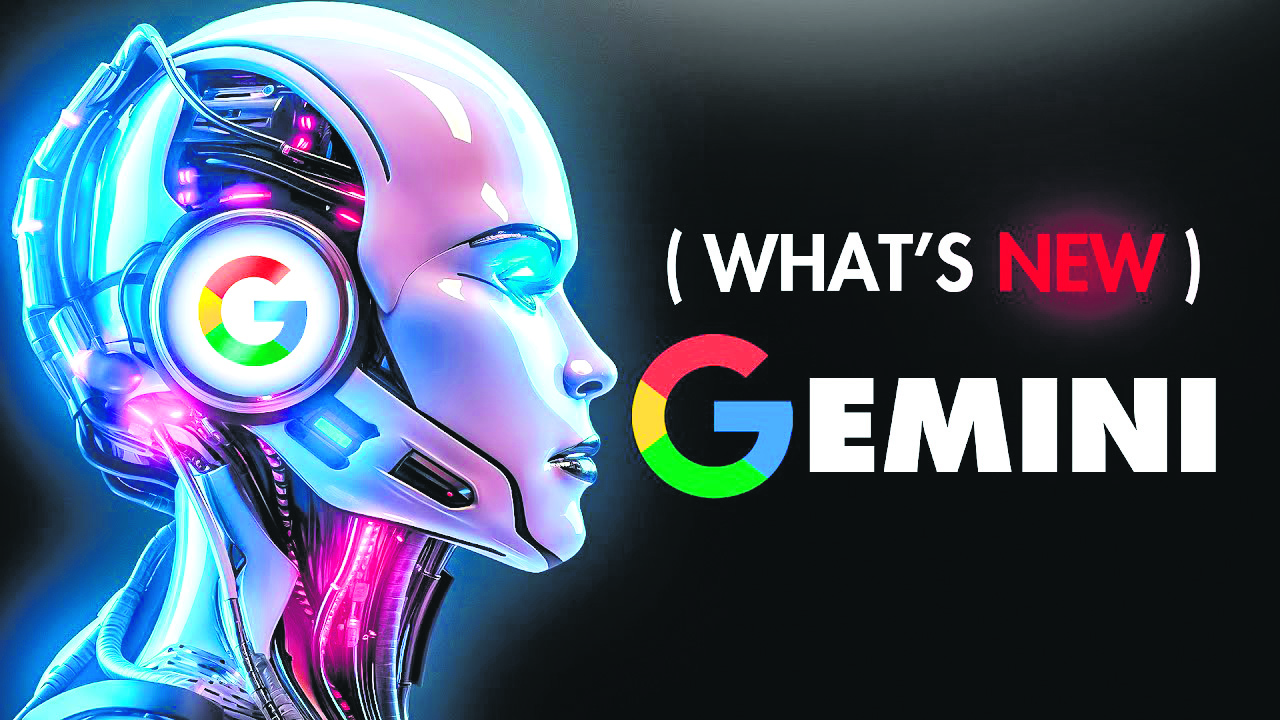
Explanation of Multimodal GEMINI AI
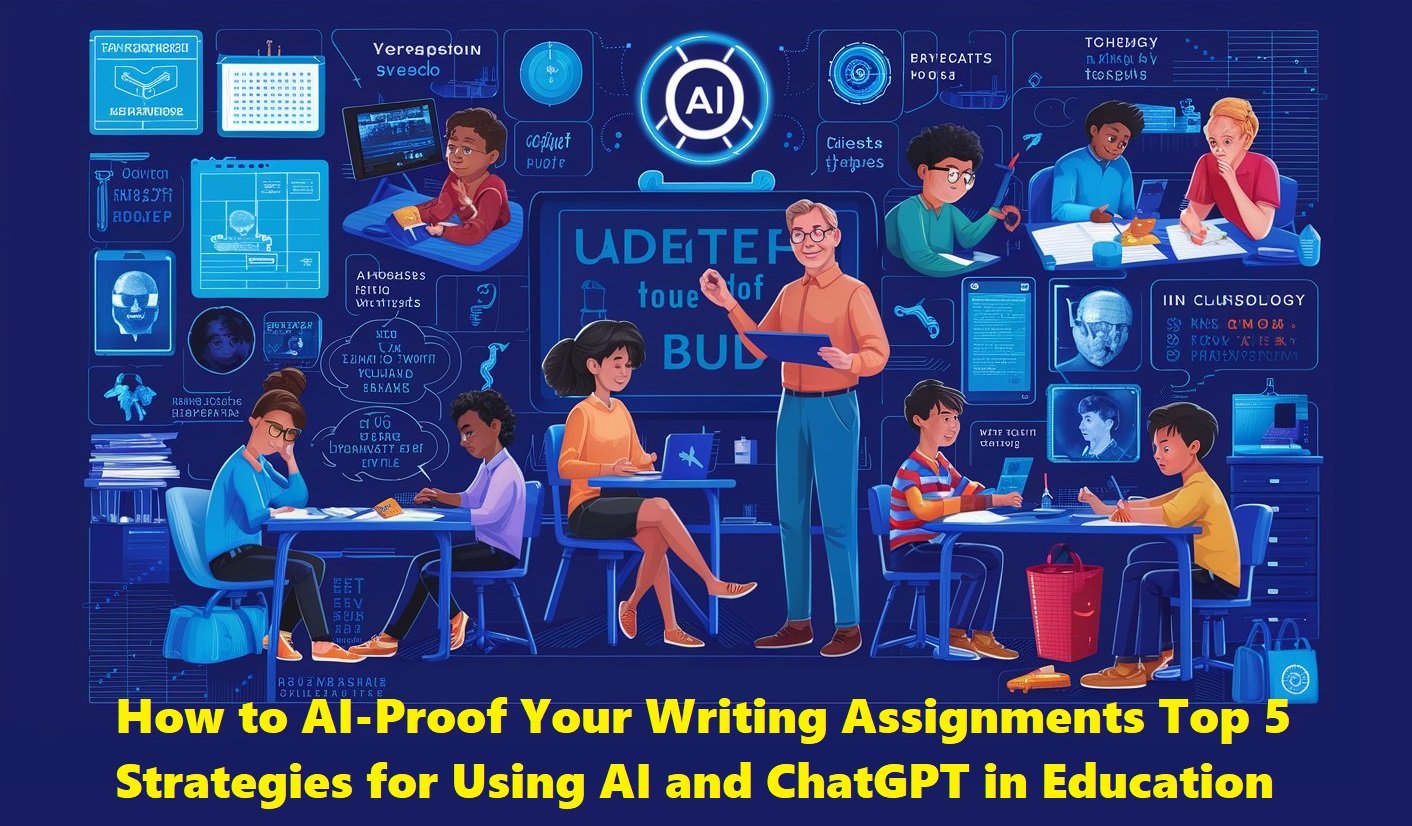
How to AI-Proof Your Writing Assignments: Top 5 Powerful Strategies for Using AI and ChatGPT in Education

Top 5 Best Programming Languages In-Demand to Skyrocket Your Career in 2024
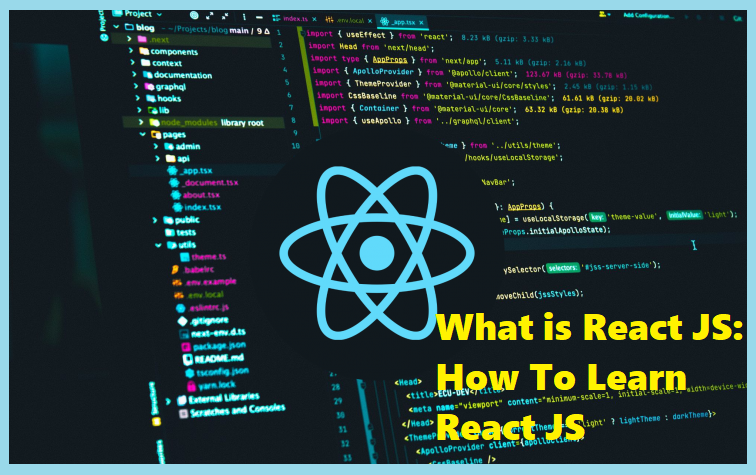
What is React JS: How To Learn React JS in 2024
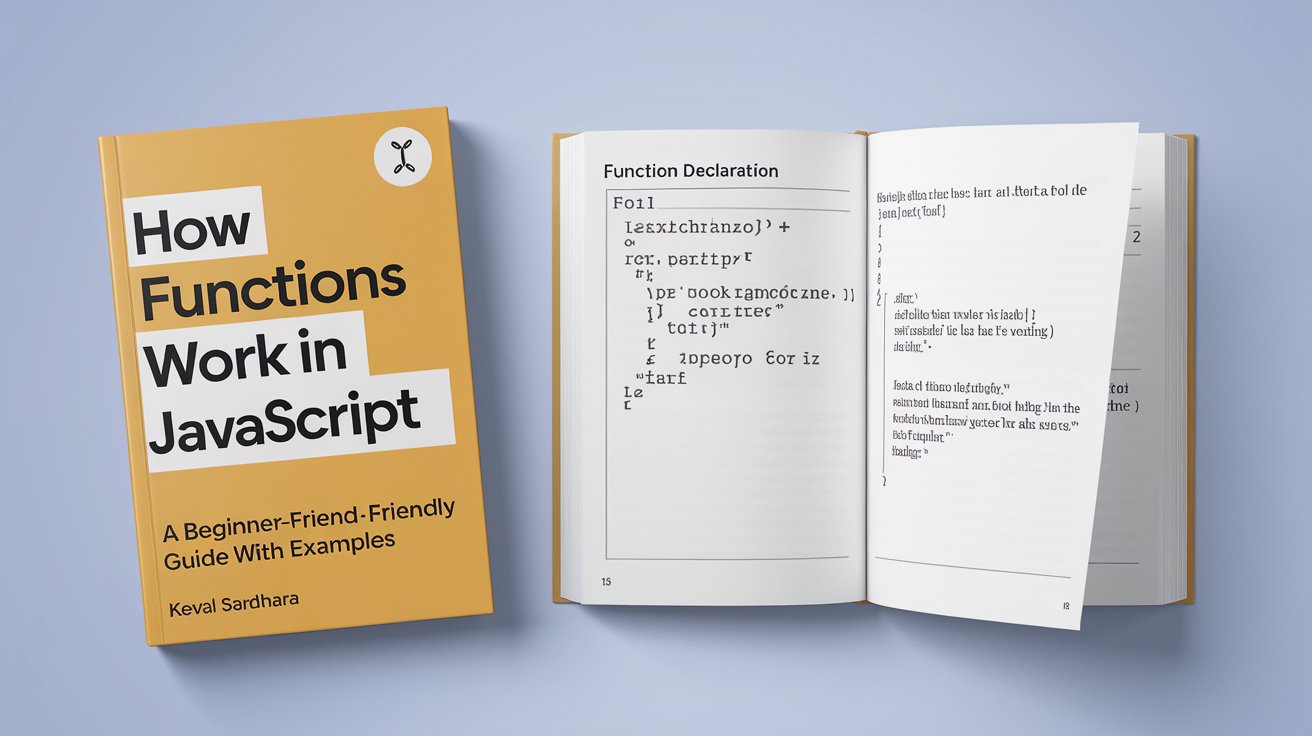
How Functions Work in JavaScript: A Beginner-Friendly Guide with Examples

Transforming the Future of Google Search: OpenAI’s SearchGPT Revolution
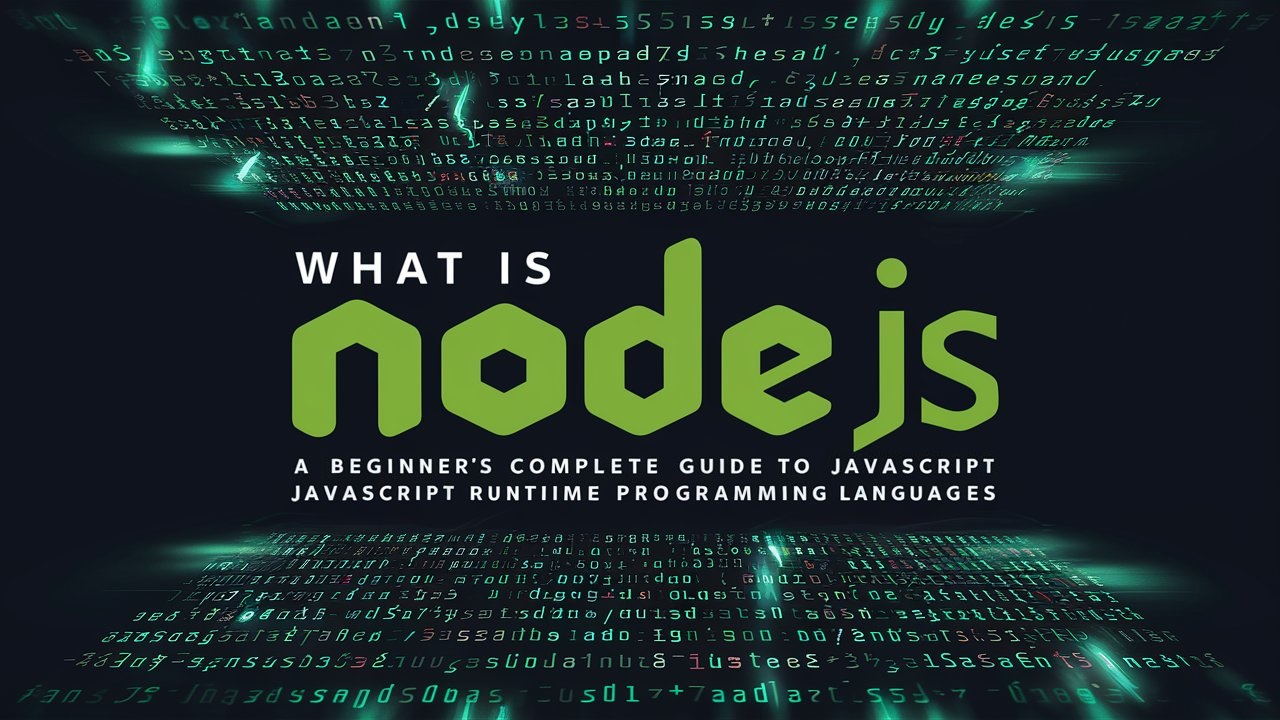