Introduction to JavaScript Functions
JavaScript is a powerful, high-level programming language widely used for web development. One of its core features is the use of functions, which are essential building blocks that help you organize code, make it reusable, and keep it maintainable. This guide is designed to help beginners understand how functions work in JavaScript, complete with examples and best practices.
Basic Function Syntax
In JavaScript, a function is a fundamental block of code designed to execute a specific task. Functions can accept input through parameters and may return a value or result. The syntax for creating a function in JavaScript is straightforward, making it an essential concept for developers to master. Understanding how to define, call, and utilize functions is crucial for writing efficient and reusable code, which is key to optimizing performance and maintainability in web development.
Example:
function functionName(parameters) {
// Function body
return result;
}
function addNumbers(a, b) {
return a + b;
}
console.log(addNumbers(5, 3)); // Outputs: 8
In this example, addNumbers
is a function that takes two parameters a
and b
, adds them, and returns the result.
Types of Functions in JavaScript
JavaScript provides multiple ways to define and use functions. Understanding these different types will enhance your coding flexibility and efficiency.
Function Declaration
A function declaration in JavaScript is a way to define a function with a given name and specified parameters. It’s a crucial concept for writing efficient and organized code. One of the key features of function declarations is hoisting, which means the function is loaded into memory during the compilation phase. Because of this, you can call the function before it actually appears in your code, making your scripts more flexible and easier to manage.
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet('Alice'); // Outputs: Hello, Alice!
Function Expression
A function expression in JavaScript is a method for creating a function and assigning it to a variable. Unlike function declarations, function expressions are not hoisted. This means you cannot call a function expression before it is defined in your code. Function expressions are useful for creating anonymous functions or assigning functions as values to variables, making them flexible and powerful tools in JavaScript programming.
const multiply = function(a, b) {
return a * b;
};
console.log(multiply(4, 5)); // Outputs: 20
Arrow Functions
Arrow functions in JavaScript provide a concise and modern syntax for writing functions. They are always anonymous, meaning they do not have a name, and are ideal for simple operations and callbacks. Arrow functions also have a shorter syntax compared to traditional function expressions, making your code cleaner and more readable. Additionally, they do not have their own this
context, which can be beneficial in certain situations, like when dealing with event handlers or array methods.
const subtract = (a, b) => a - b;
console.log(subtract(10, 3)); // Outputs: 7
Arrow functions in JavaScript don’t have their own this
context. Instead, they inherit this
from the surrounding scope. This is especially helpful in callbacks and methods where keeping the correct this
reference is important.
Example:
function Person() {
this.age = 0;
setInterval(() => {
this.age++; // 'this' refers to the Person object
}, 1000);
}
In this example, the arrow function ensures that this
refers to the Person
object, avoiding issues with this
inside the callback.
Anonymous Functions
Anonymous functions are functions without a name. They are commonly used as arguments in other functions or in immediately invoked function expressions (IIFE).
// Using an anonymous function as an argument
setTimeout(function() {
console.log('This message appears after 1 second');
}, 1000);
// Immediately Invoked Function Expression (IIFE)
(function() {
console.log('This function runs immediately');
})();
Named Functions
A named function expression is similar to an anonymous function but includes a name. This naming is particularly useful for recursion and enhances debugging, as it provides a clear reference to the function.
// Named function expression
const factorial = function calculateFactorial(n) {
if (n === 0) {
return 1;
}
return n * calculateFactorial(n - 1); // Recursive call
};
console.log(factorial(5)); // Output: 120
Constructor Functions
Constructor functions are used to create objects. They are defined with a capitalized name and invoked using the new
keyword.
function Person(name, age) {
this.name = name;
this.age = age;
}
const person1 = new Person('Bob', 25);
console.log(person1.name); // Outputs: Bob
Callback Functions
Callback functions are functions passed as arguments into other functions and are executed within those functions. They are essential for handling asynchronous operations and managing tasks that need to be performed after certain events occur.
function fetchData(callback) {
setTimeout(() => {
const data = { name: 'Alice', age: 30 };
callback(data);
}, 2000);
}
function displayData(data) {
console.log(`Name: ${data.name}, Age: ${data.age}`);
}
fetchData(displayData);
// After 2 seconds, Outputs: Name: Alice, Age: 30
Closures in JavaScript
Closures are functions that retain access to their own scope, the outer function’s scope, and the global scope. This feature allows functions to encapsulate data and maintain state.
function createCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
In this example, the inner function has access to the count
variable defined in createCounter
, even after createCounter
has finished executing.
Higher-Order Functions
Higher-order functions are functions that either take other functions as arguments or return functions as their result. They are central to functional programming in JavaScript.
// Function that takes another function as an argument
function applyOperation(x, operation) {
return operation(x);
}
// Function to be used as a callback
function square(n) {
return n * n;
}
// Using a higher-order function
console.log(applyOperation(5, square)); // Output: 25
// Function that returns another function
function makeMultiplier(factor) {
return function(number) {
return number * factor;
};
}
const double = makeMultiplier(2);
console.log(double(4)); // Output: 8
Default Parameters in JavaScript
Default parameters in JavaScript let you set default values for function parameters. If a value is not provided or is undefined
, the default value is used.
function greet(name = 'Guest') {
return `Hello, ${name}!`;
}
console.log(greet()); // Output: Hello, Guest!
console.log(greet('Alice')); // Output: Hello, Alice!
JavaScript Built-in Functions
JavaScript offers a variety of built-in functions for performing everyday tasks. Here are some frequently used ones:
parseInt()
: Converts a string to an integer.parseFloat()
: Converts a string to a floating-point number.isNaN()
: Checks if a value isNaN
(Not a Number).Array.isArray()
: Determines if a value is an array.
console.log(parseInt('42')); // Outputs: 42
console.log(parseFloat('3.14')); // Outputs: 3.14
console.log(isNaN('Hello')); // Outputs: true
console.log(Array.isArray([1, 2])); // Outputs: true
Conclusion
Functions are essential building blocks in JavaScript programming. They enable you to decompose complex problems into smaller, more manageable pieces, leading to cleaner, more organized, and reusable code. Mastering various types of functions—such as function declarations, function expressions, arrow functions, and higher-order functions—will significantly boost your ability to write efficient and effective JavaScript code. By understanding these different function types, you’ll be well-equipped to tackle a wide range of programming challenges and enhance your overall coding skills.
Additional Resources
- MDN Web Docs on Functions
- JavaScript Functions Tutorial – W3Schools
- Understanding JavaScript Function Expressions – GeeksforGeeks
- JavaScript Arrow Functions Explained – FreeCodeCamp
FAQs
What is the difference between a function declaration and a function expression?
Function declaration defines a named function and is hoisted so it can be called before it’s defined in the code. A function expression defines a function as part of a variable assignment and is not hoisted.
What are arrow functions in JavaScript?
Arrow functions are a concise syntax for writing functions using the =>
notation. They are always anonymous and do not have their own this
context.
How do closures work in JavaScript?
Closures are functions that retain access to their lexical scope even when executed outside of it. This allows the function to access variables from its parent scope.
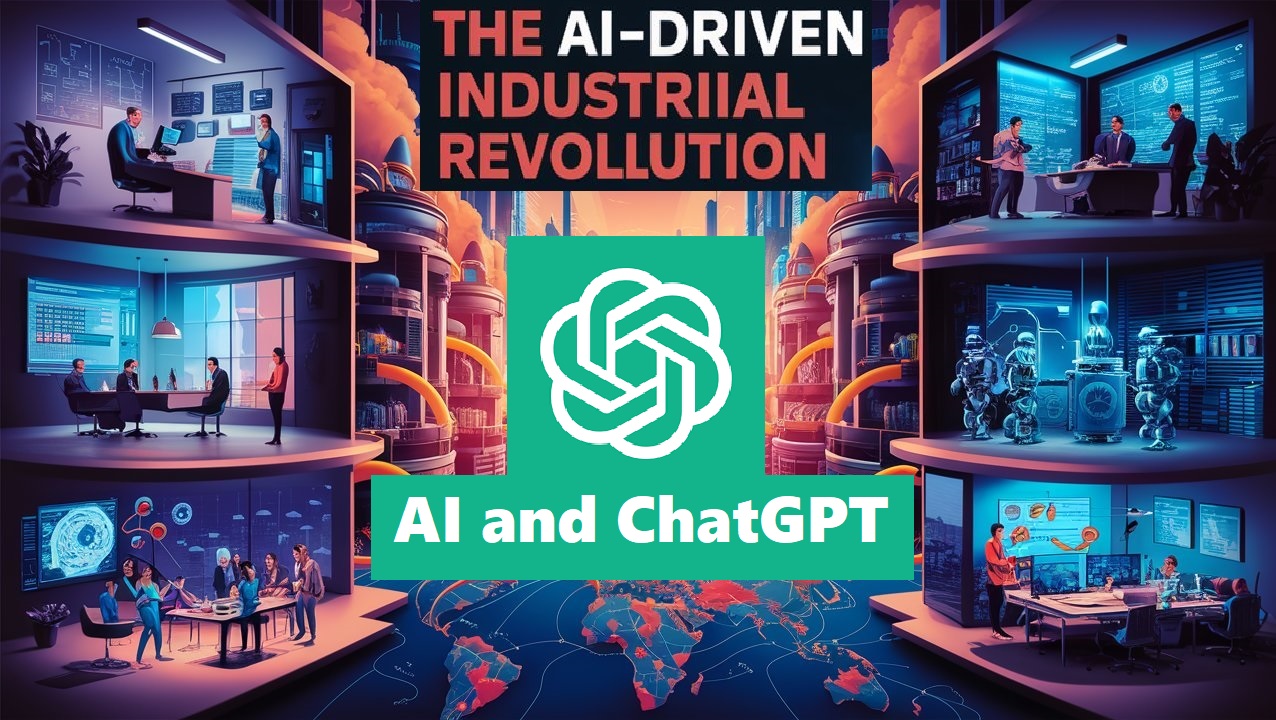
How AI and ChatGPT Technology Transform the Next Industrial Revolution
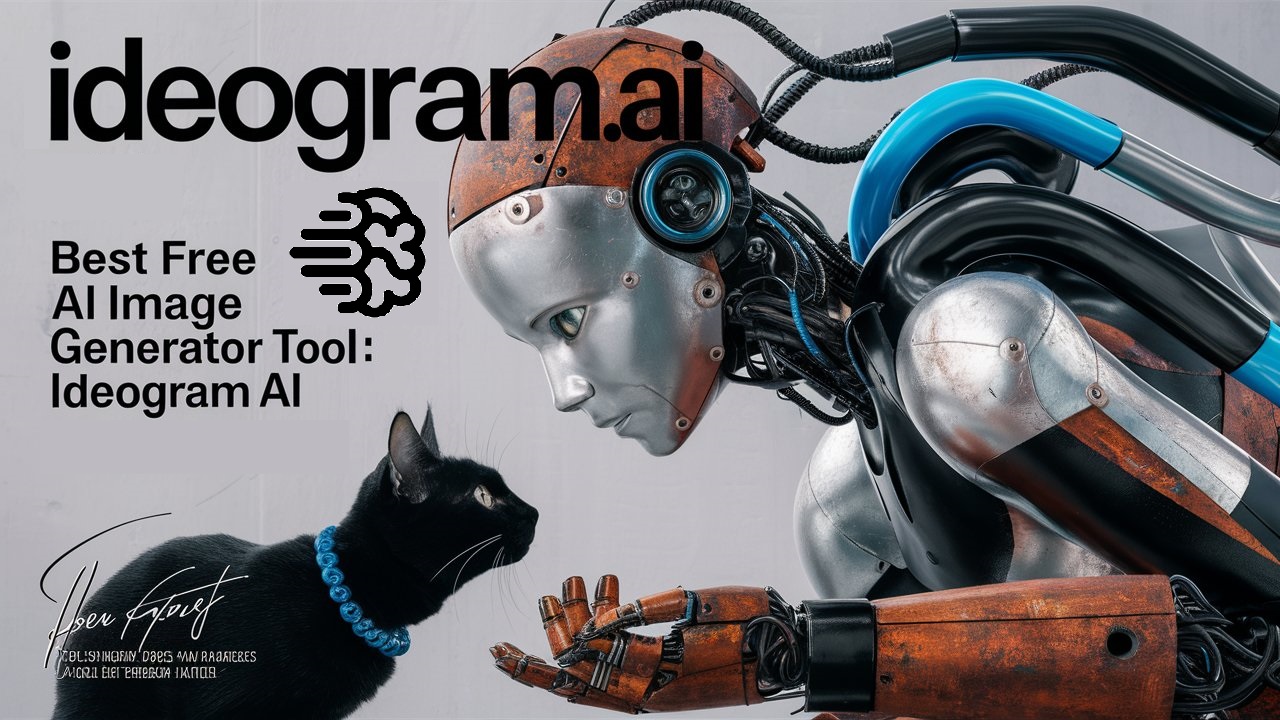
Best Free AI Image Generator Tool: Ideogram AI
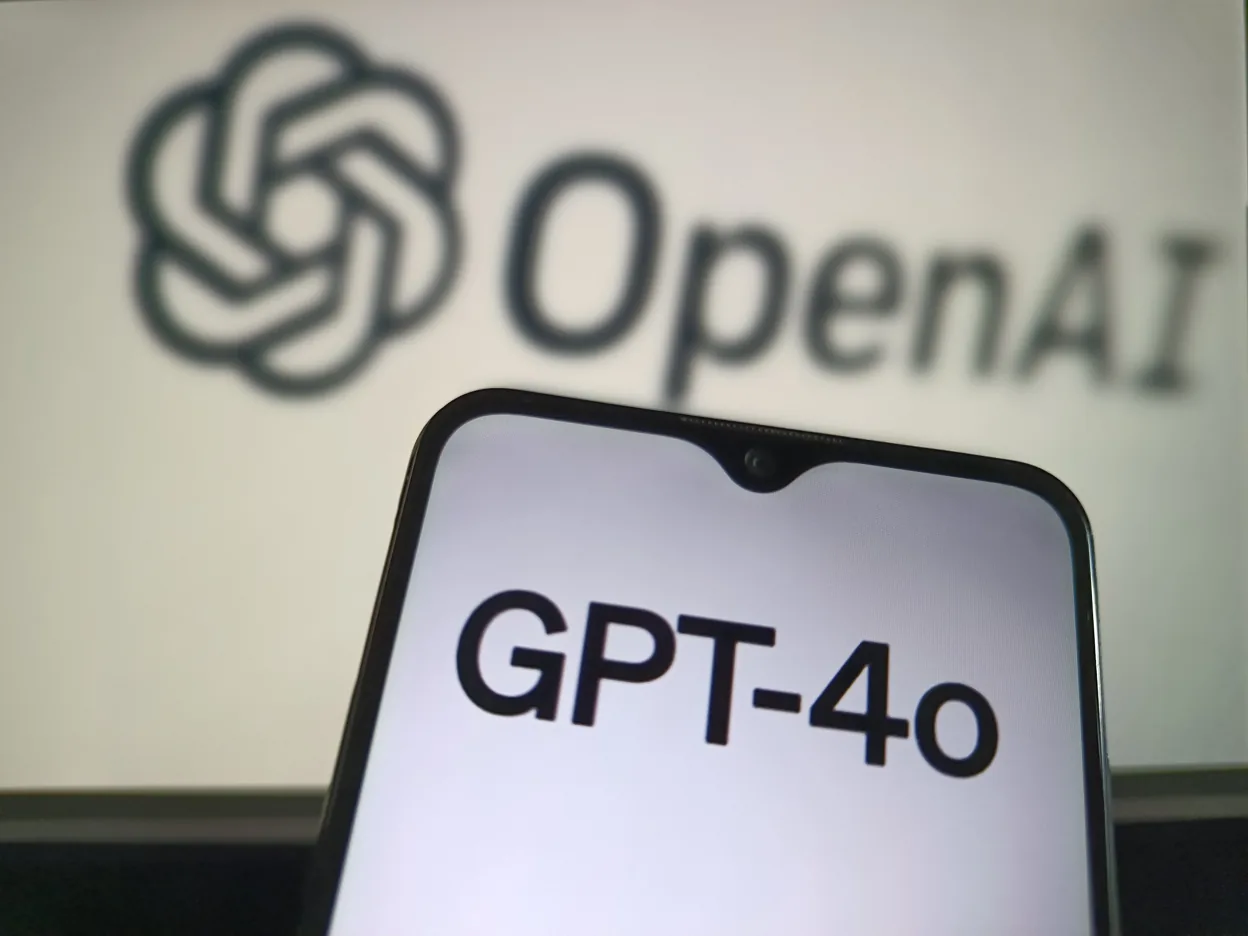
GPT-4o: How ChatGPT-Integrates Audio, Vision, and Text
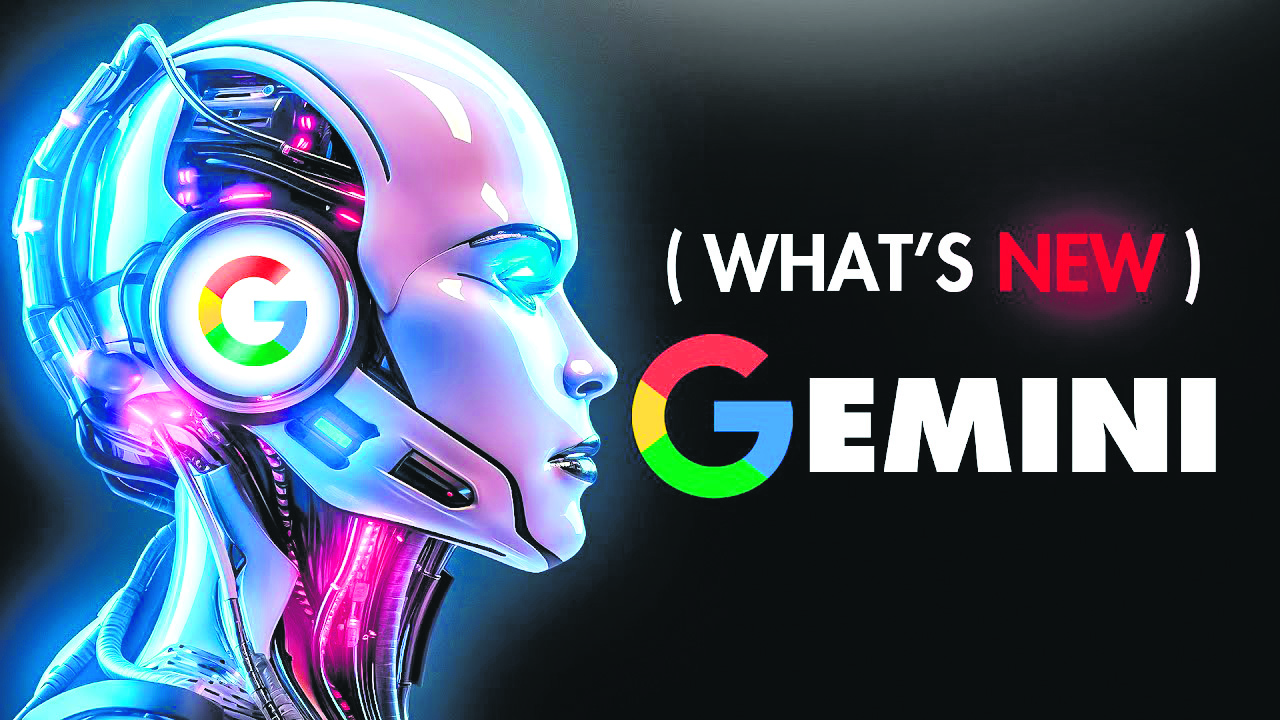
Explanation of Multimodal GEMINI AI
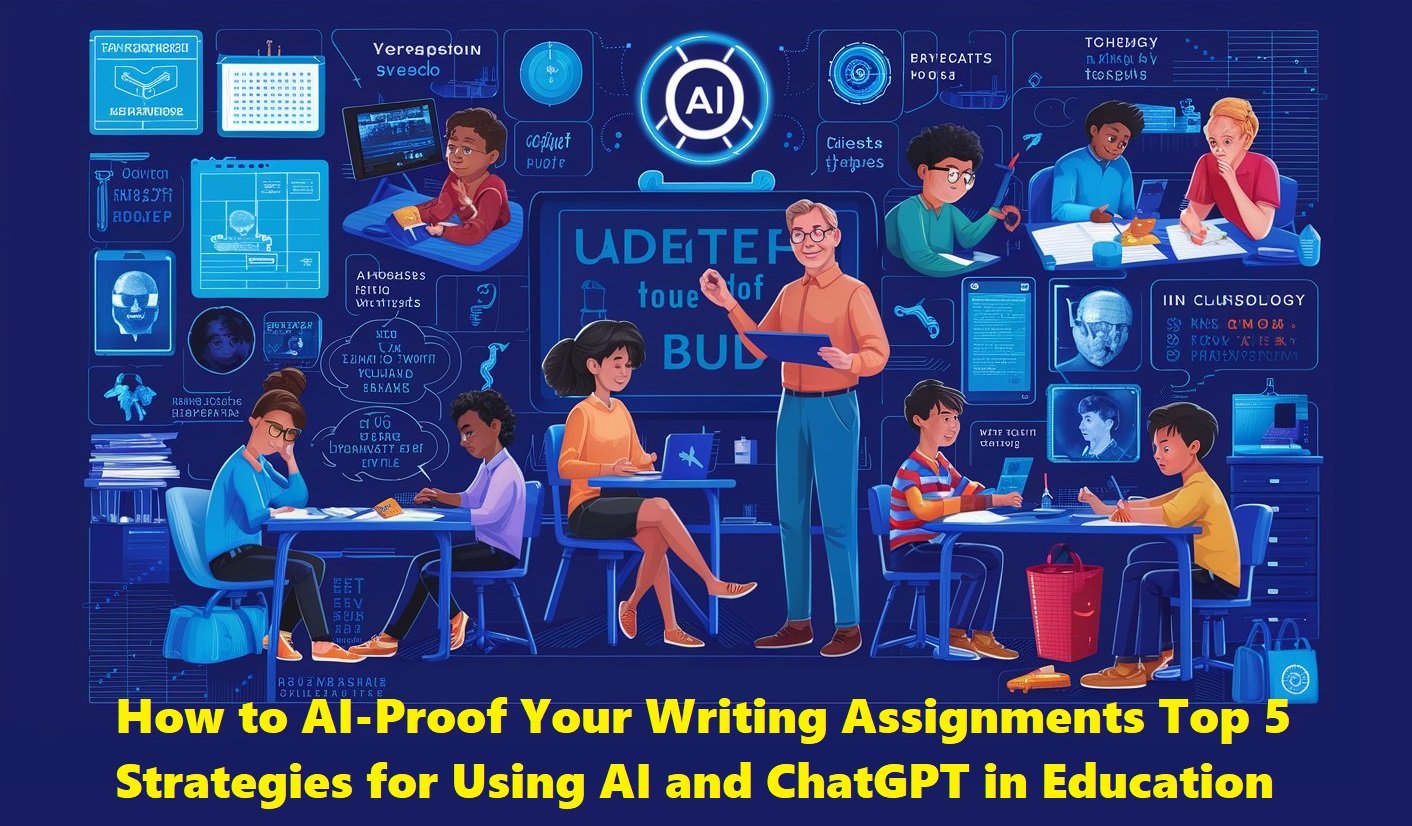
How to AI-Proof Your Writing Assignments: Top 5 Powerful Strategies for Using AI and ChatGPT in Education

Top 5 Best Programming Languages In-Demand to Skyrocket Your Career in 2024
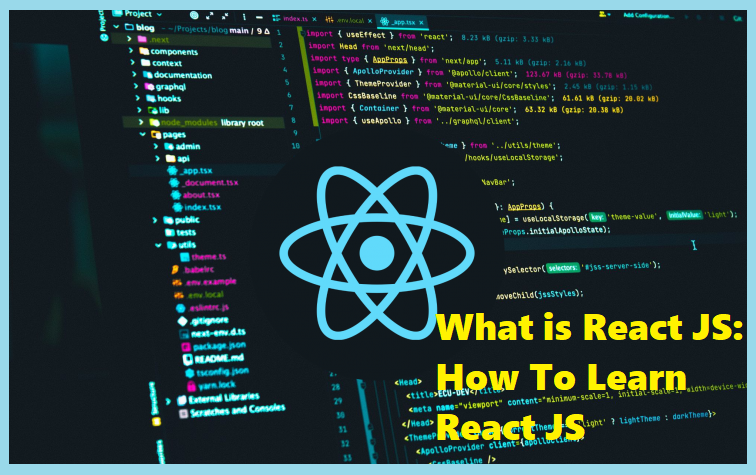
What is React JS: How To Learn React JS in 2024
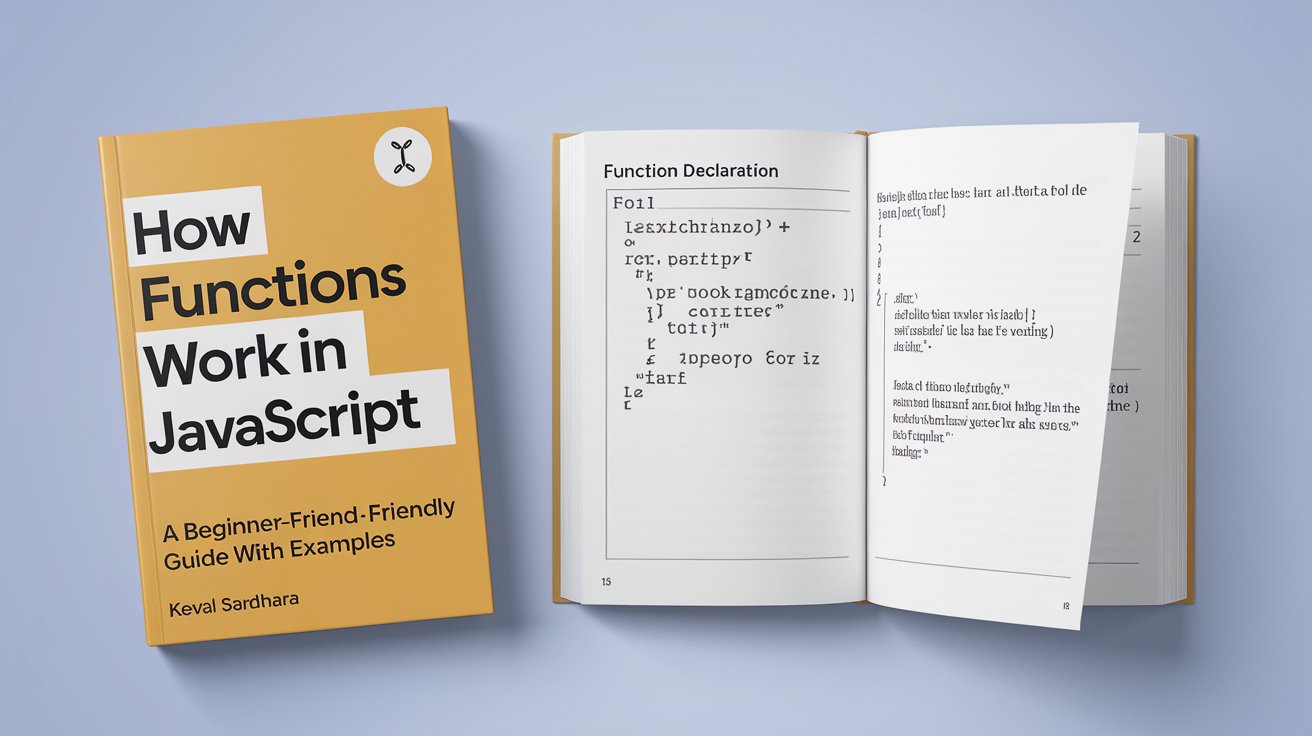
How Functions Work in JavaScript: A Beginner-Friendly Guide with Examples

Transforming the Future of Google Search: OpenAI’s SearchGPT Revolution
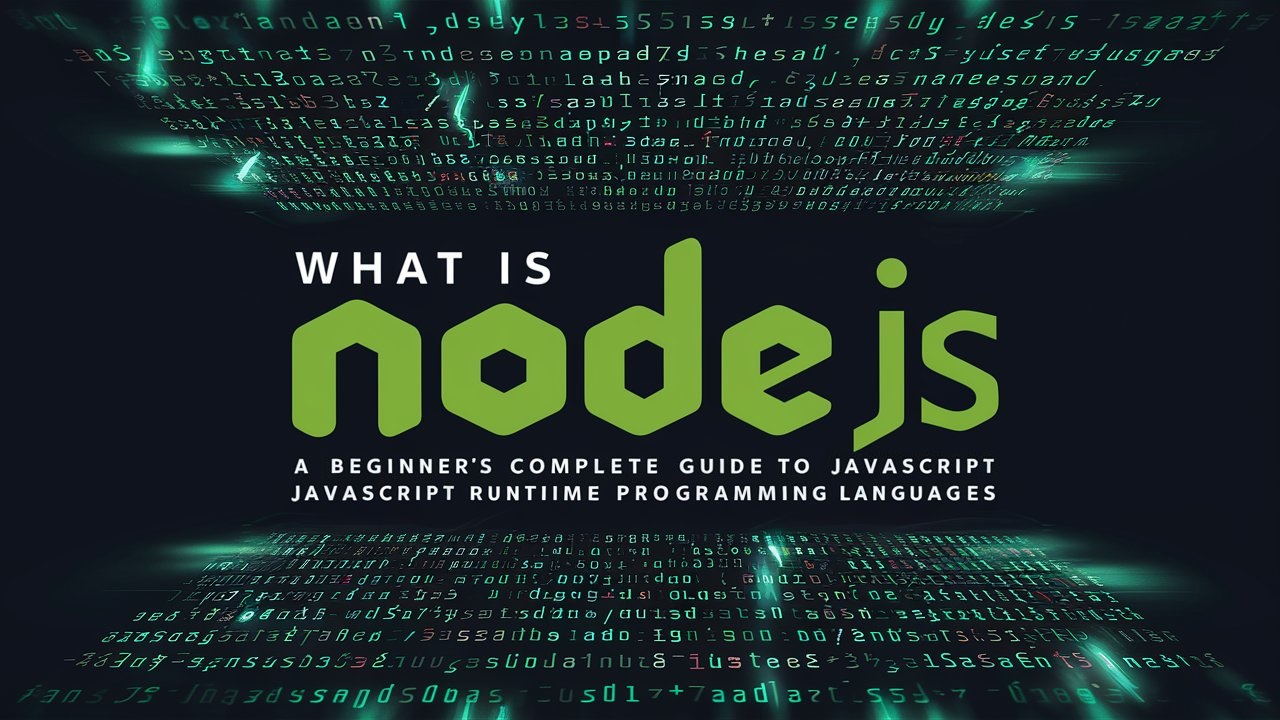
What is Node.js? A Beginner’s Complete Guide to Learn JavaScript Runtime Server-Side Programming Languages
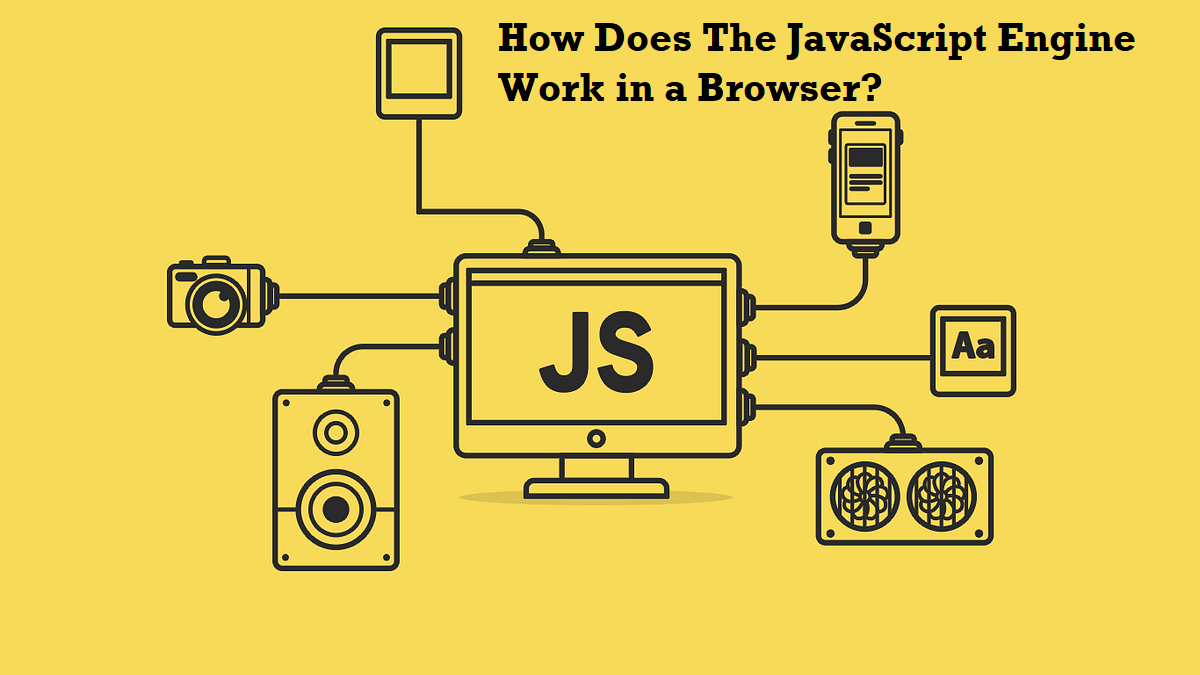
How JavaScript Works Behind the Scene? JS Engine and Runtime Explained
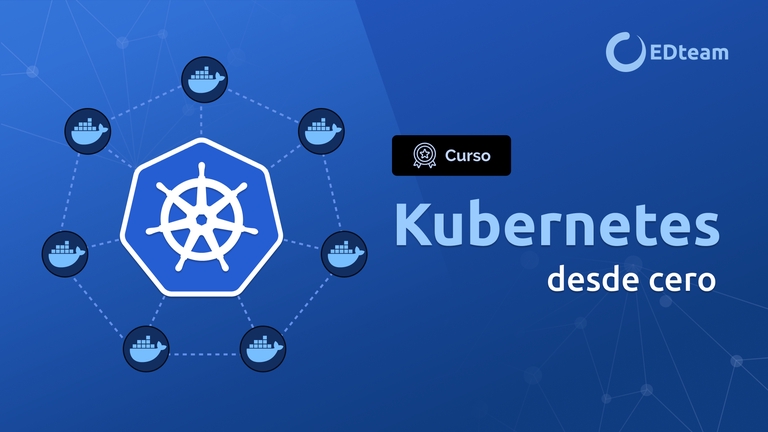
How to master Kubernetes Architecture: A Simple Guide for Enhanced Operations
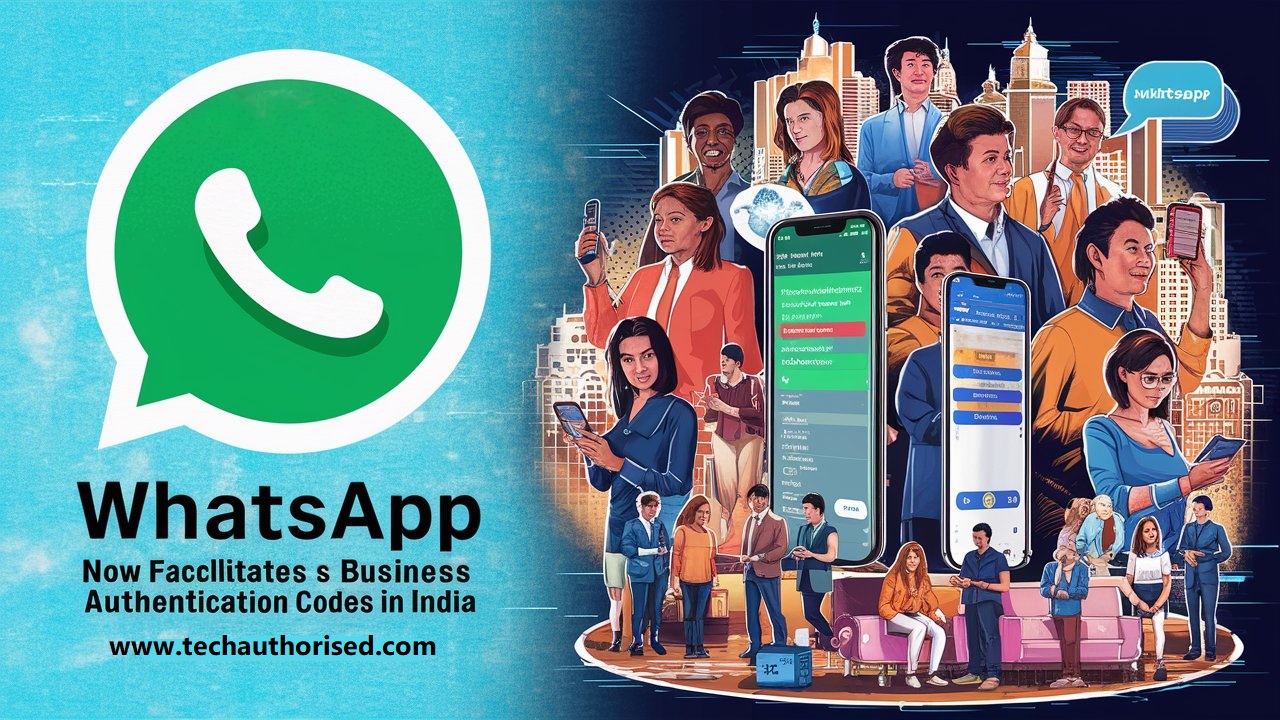
WhatsApp Now Facilitate Business Authentication Code in India
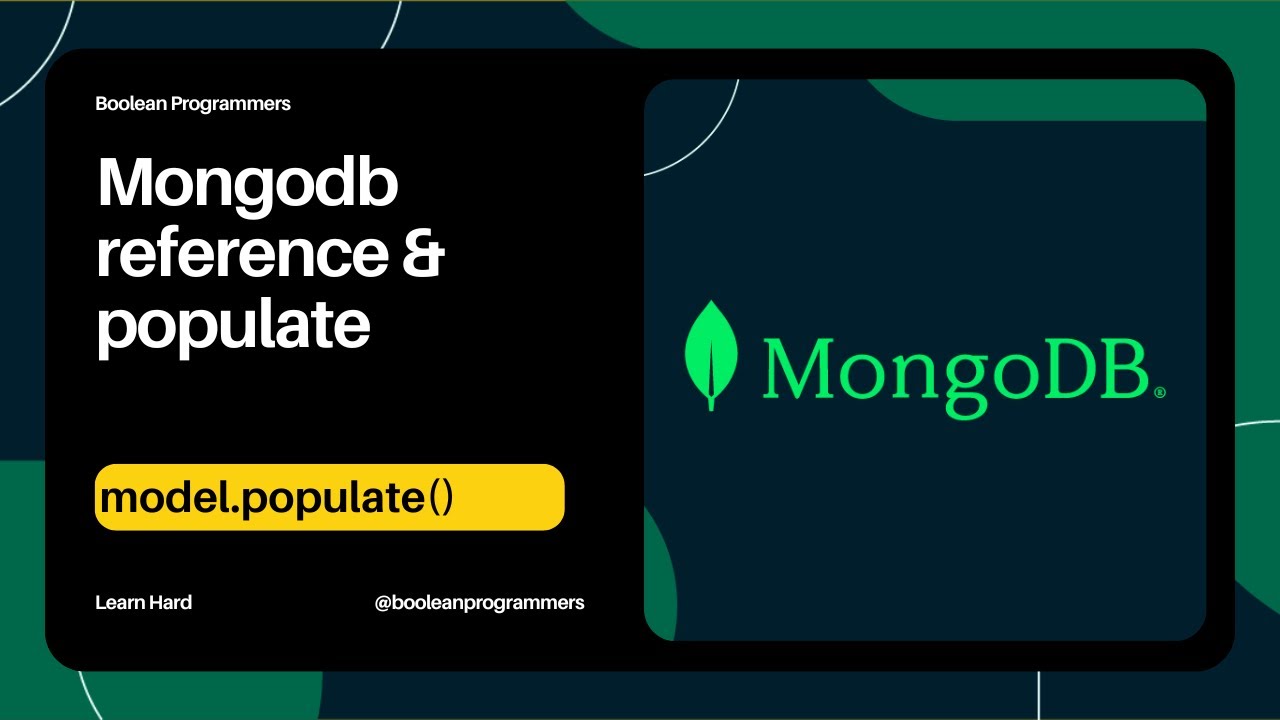
How to work the ‘populate’ Method in Mongoose: Simple Guide to Understanding More Complex MongoDB Query
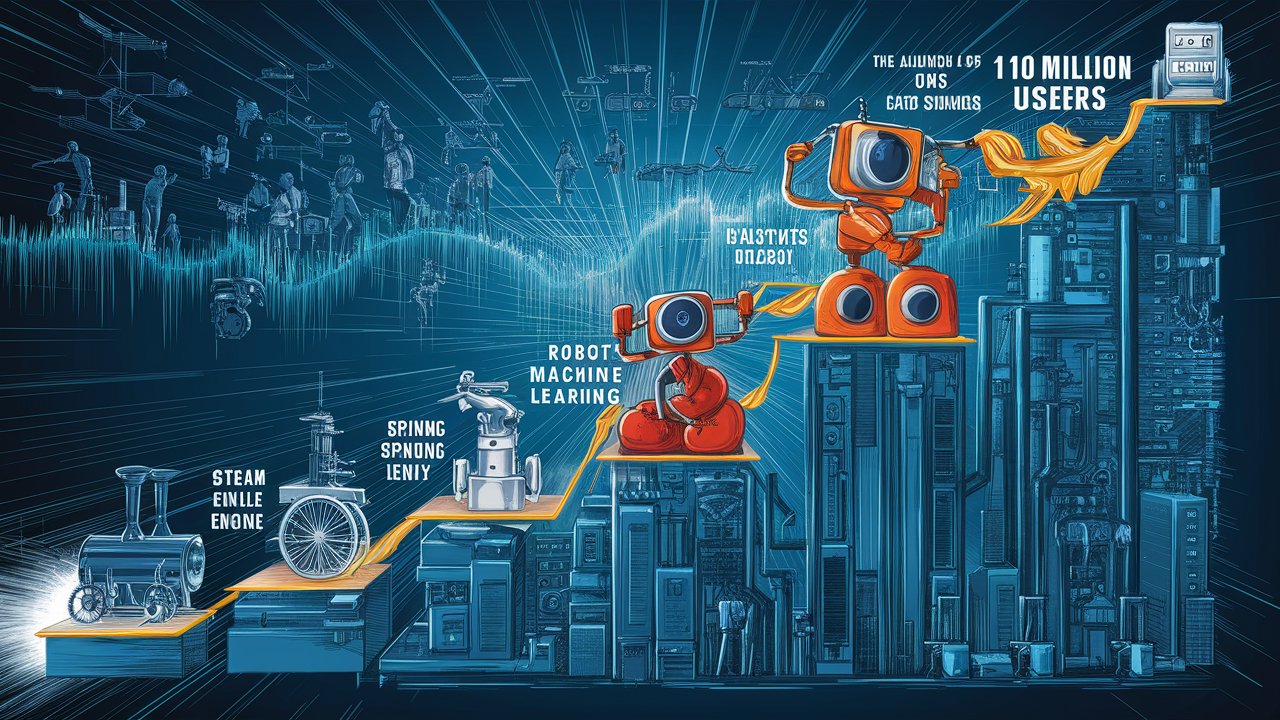