JavaScript is a highly popular programming language that continues to grow in power over time. It is a simple, object-oriented, platform-independent language made specifically for managing browser data. It provides several libraries for various tasks, making it a versatile tool in web development.
JavaScript applications are often referred to as “WORA” (Write Once, Run Anywhere) applications because this programming language enables code execution on any platform or browser without altering the original script. Due to its growing popularity, numerous businesses utilize it across their technology stacks, including the front-end, back-end, full-stack, and embedded apps.
This article will help you understand how JavaScript works Behind the Scene? internally before diving deeply into JavaScript programming. We’ll explore and understand how JavaScript works from the inside out.
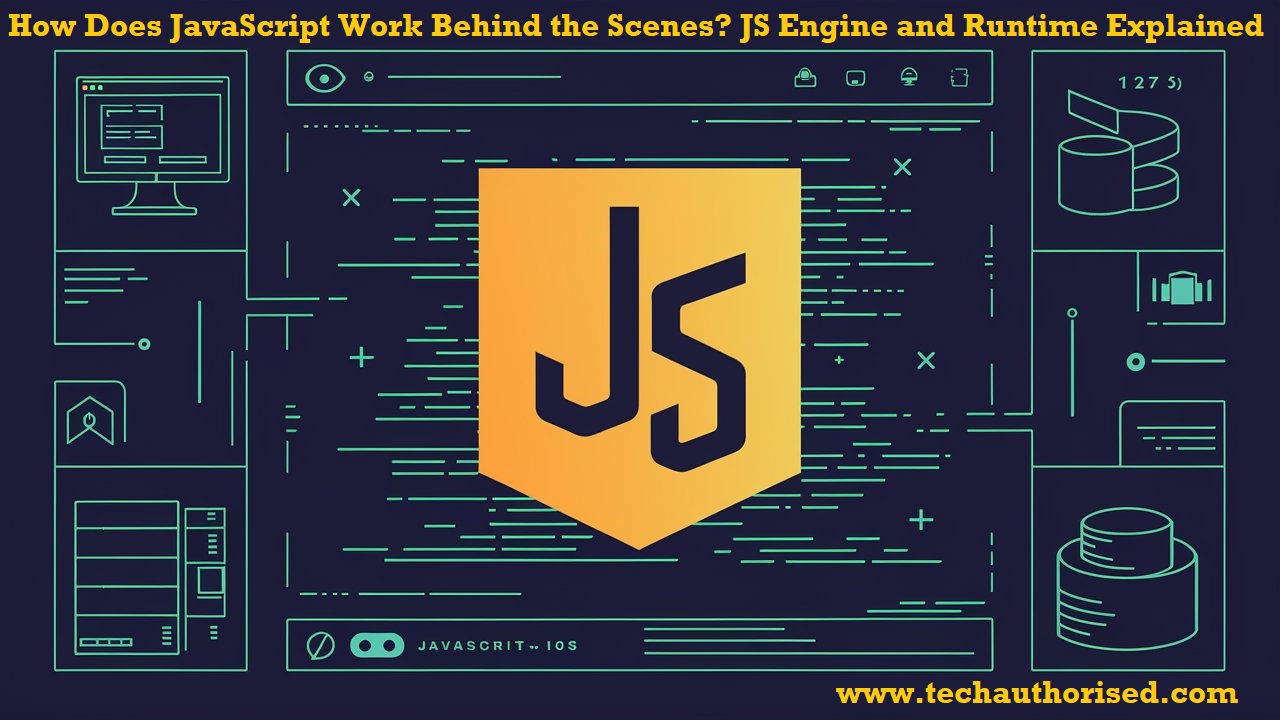
JavaScript Basics
JavaScript is a single-threaded programming language, meaning that only one command is executed at a time. This makes JavaScript blocking and synchronous in nature, where code runs in the order it appears, and each section must be completed before moving on to the next. However, JavaScript can still execute applications asynchronously and without blocking the main thread.
Type checking in JavaScript happens in real time because it is a dynamically typed language. This means that when you declare a variable, you don’t need to specify its type. You simply use var
, let
, or const
to declare variables, and JavaScript will handle the rest.
Basic Javascript Syntex
// Variables
let name = "techauthorised.com"; // String
const age = 30; // Number
let isDeveloper = true; // Boolean
// Array
let skills = ["JavaScript", "Python", "C++"];
// Object
let person = {
name: "Keval",
age: 30,
isDeveloper: true,
skills: ["JavaScript", "Python", "C++"]
};
// Function
function greet(personName) {
return `Hello, ${personName}!`;
}
// Conditional Statements
if (isDeveloper) {
console.log(greet(name)); // Output: Hello, all Student
}
// Loops
for (let i = 0; i < skills.length; i++) {
console.log(`Skill ${i + 1}: ${skills[i]}`);
}
// Functions with Return Value
function add(a, b) {
return a + b;
}
let sum = add(5, 10);
console.log(`Sum: ${sum}`); // Output: Sum: 15
// Arrow Function
const multiply = (x, y) => x * y;
let product = multiply(4, 5);
console.log(`Product: ${product}`); // Output: Product: 20
// String Methods
let message = "Welcome to JavaScript!";
console.log(message.toUpperCase()); // Output: WELCOME TO JAVASCRIPT!
// Object Methods
person.sayHello = function() {
return `Hi, I am ${this.name}.`;
}
console.log(person.sayHello()); // Output: Hi, All Welcome to techauthorised.com
Components of JavaScript
JavaScript Engine
JavaScript is an interpreted programming language, which means it doesn’t need to be compiled into binary code before execution. The JavaScript engine is responsible for executing JavaScript code by interpreting it directly.
When a JavaScript file is loaded into the browser, the JavaScript engine runs each line of code sequentially, from top to bottom. It translates each line into binary code, which the browser can then execute. Each browser has its own JavaScript engine. Here are some examples:
- Google Chrome: V8
- Safari: JavaScriptCore
- Mozilla Firefox: SpiderMonkey
- Microsoft Edge: Chakra
JavaScript engine has two main components:
- Memory Heap: An unstructured memory pool that stores objects and variables.
- Call Stack: The place where code execution takes place. It keeps track of where we are in the code.
How JavaScript Works Behind the Scene? Runtime JS Engine
The JavaScript engine does not work alone; it operates within the JavaScript Runtime Environment (JRE). The JRE includes several components that allow JavaScript to be asynchronous and interact with other parts of the browser.
Components of the JRE include:
- JavaScript Engine: Executes JavaScript code.
- Web API: Provides APIs for tasks like DOM manipulation, HTTP requests, and timers.
- Callback Queue: Holds messages to be processed.
- Event Loop: Continuously checks the call stack and callback queue, executing tasks in the queue when the call stack is empty.
- Event Table: Maintains records of events and their associated callback functions.
JavaScript Call Stack
Call stack is a data structure that keeps track of the execution of function calls. JavaScript being single-threaded means it can only do one thing at a time. The call stack helps manage the execution order of functions.
When a function is called, it is pushed onto the stack. When the function returns a result, it is popped off the stack. If a function calls another function, the new function is pushed onto the top of the stack.
JavaScript Concurrency Model and Event Loop
JavaScript can handle multiple tasks simultaneously, thanks to the event loop. The event loop allows JavaScript to perform non-blocking operations despite its single-threaded nature. Here’s how it works:
- Call Stack: Where your JavaScript code runs.
- Web APIs: Handle asynchronous operations like HTTP requests.
- Callback Queue: Holds messages to be processed by the event loop.
- Event Loop: Monitors the call stack and callback queue, moving tasks from the queue to the stack when the stack is empty.
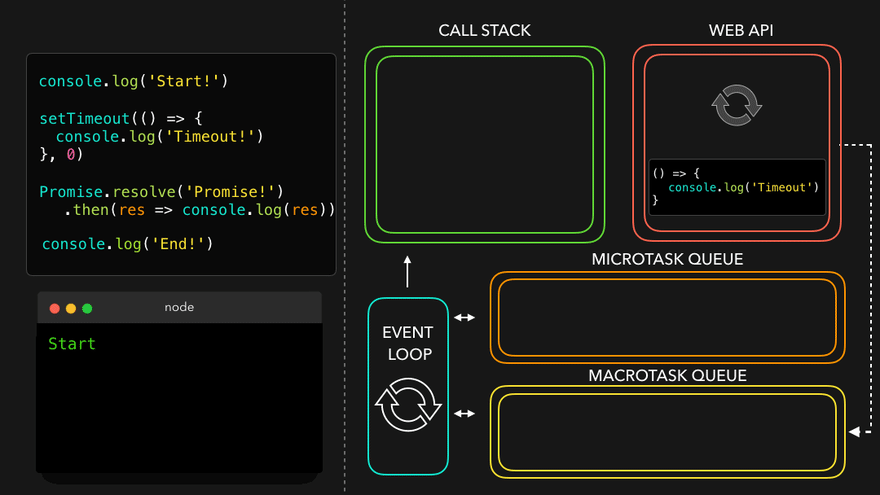
This model prevents the browser from becoming unresponsive by efficiently managing tasks.
How Does JavaScript Engine Work?
Environment
JavaScript code runs within specific environments, the most common being browsers and Node.js. These environments contain engines that translate human-readable code into machine code that computers can execute.
Engine
Each environment includes an engine, like Chrome’s V8, Firefox’s SpiderMonkey, Safari’s JavaScriptCore, and IE’s Chakra. These engines have similar functionality but may differ in implementation. The engine’s primary role is to take human-readable code and translate it into machine code.
Parser
The parser is a component of the engine that reads the code line by line, checking for syntax errors. If the code is valid, the parser generates an Abstract Syntax Tree (AST), a structured representation of the code.
Explore how JavaScript works behind scenes with Akshay Saini’s popular YouTube series, Namaste JavaScript. This playlist explains the details of JavaScript, like the JS engine, runtime, execution contexts, hoisting, scopes, closures, and the event loop. Akshay’s clear and simple explanations, along with helpful visuals and code examples, make learning easy. Loved by many, this series is perfect for anyone who wants to get better at JavaScript. Watch now and improve your JavaScript Programming skills!
For more, visit us: https://www.youtube.com/watch?v=pN6jk0uUrD8&list=PLlasXeu85E9cQ32gLCvAvr9vNaUccPVNP
Abstract Syntax Tree (AST)
The AST is a tree representation of the code that the parser generates. It breaks down the code into its fundamental components, making it easier for the engine to process and execute.
For more Information related to Abstract Syntax Tree (AST) visit us: https://astexplorer.net/
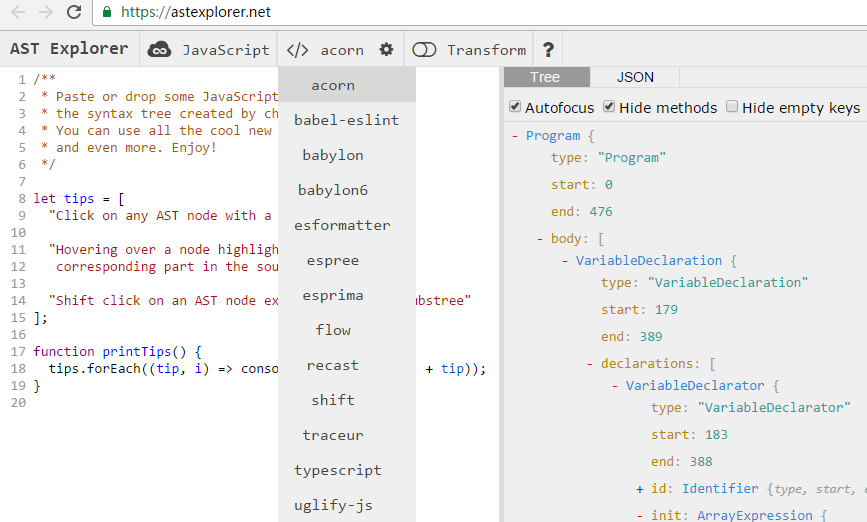
Interpreter and Compiler
The interpreter takes the AST and converts it into an Intermediate Representation (IR). The compiler then takes this IR and optimizes it, converting it into machine code that the processor can execute. JavaScript engines often use Just-In-Time (JIT) compilation, which combines interpretation and compilation for efficiency.
Execution Contexts and Execution Stack
An execution context is an abstract concept that holds information about the environment within which the current code is being executed. There are two types of execution contexts in JavaScript:
- Global Execution Context (GEC): The default context where all non-function code runs. There is only one GEC per program.
- Functional Execution Context: Created whenever a function is invoked.
Execution Stack
The execution stack, also known as the call stack, is a stack data structure that keeps track of the execution contexts. When a function is called, a new execution context is created and pushed onto the stack. When the function completes, its execution context is popped off the stack.
How Does The JavaScript Engine Execute Your Code?
When the JavaScript engine starts executing code, it creates the Global Execution Context (GEC). The GEC creation phase involves the following steps:
- Creating the Global Object: This is typically the
window
object in browsers. - Binding
this
to the Global Object: In the global context,this
refers to the global object. - Setting Up the Memory Heap: Allocating memory for variables and functions.
- Hoisting: Moving function declarations and variable declarations to the top of their scope before execution begins.
Once the GEC is set up, the engine starts executing the code line by line.
How Does The JavaScript Engine Work in a Browser?
When a JavaScript program runs in a browser, the following steps occur:
- Parsing JavaScript File: The parser reads the code line by line, checking for syntax errors. If no errors are found, it generates an AST.
- Creating Abstract Syntax Tree (AST): The AST represents the code structure.
- Converting JavaScript Code to Machine Code: The engine translates the AST into machine code.
- Executing Machine Code: The machine code is executed, producing the final output.
Conclusion
How JavaScript Works Behind the Scene? Runtime JS engines are present in most modern web browsers. When a JavaScript program is executed, the engine parses the code, creates an AST, converts it to machine code, and executes it. Understanding how JavaScript works behind the scenes helps developers write more efficient and effective code. We hope this article gave you a complete view of how JavaScript works.
By understanding these concepts, you can better appreciate the power and flexibility of JavaScript in modern web development.
Best References to Learn JavaScript in 2024
MDN Web Docs
- Website: MDN JavaScript
- Best for: Comprehensive documentation and tutorials on JavaScript, covering fundamental and advanced topics.
JavaScript.info
- Website: JavaScript.info
- Best for: Detailed and beginner-friendly explanations of JavaScript concepts with practical examples.
Eloquent JavaScript (Book)
- Website: Eloquent JavaScript
- Best for: An in-depth book that covers JavaScript from the basics to advanced topics, including programming techniques.
W3Schools
- Website: W3Schools JavaScript Tutorial
- Best for: Easy-to-follow tutorials and interactive examples for beginners learning JavaScript.
Codecademy
- Website: Codecademy JavaScript Course
- Best for: Interactive and hands-on JavaScript courses that provide immediate feedback and practical coding experience.
freeCodeCamp
- Website: freeCodeCamp JavaScript Algorithms and Data Structures
- Best for: Free and extensive curriculum covering JavaScript algorithms and data structures with practical projects.
Coursera
- Website: Coursera JavaScript Courses
- Best for: University-level courses on JavaScript taught by experts from top institutions, offering structured learning paths.
■ Explore More About TechAuthorised
-
In today’s fast-paced business world, innovation drives industries forward. We are now at the dawn of the AI-driven Industrial Revolution. This new era, characterized by the convergence of artificial intelligence (AI) and advanced language models like ChatGPT, is transforming how companies operate, communicate, and compete. Unlike previous industrial revolutions, which were limited to specific sectors, AI and ChatGPT are impacting every aspect of…
-
Ideogram (pronounced “eye-diogram”) is a new AI company on a mission to help people become more creative. The company is developing state-of-the-art AI tools that will make creative expression more accessible, fun, and efficient. It’s pushing the limits of what’s possible with AI, focusing on creativity and maintaining high standards for trust and safety.
-
GPT-4o accepts any combination of text, audio, image, and video as input and can generate outputs in text, audio, and image formats. This flexibility allows for more natural and intuitive interactions. For instance, it can respond to audio inputs in as little as 232 milliseconds, which is close to human response time in a conversation.
-
In an era where artificial intelligence is becoming integral to various aspects of life, Google Gemini stands out for its multimodal capabilities, which allow it to process and interpret text, audio, images…
-
Artificial Intelligence (AI) has revolutionized many fields, including education. While AI offers countless benefits, it poses significant challenges, particularly regarding academic integrity. With the advent of AI-enabled tools like ChatGPT, there is a growing concern about cheating and plagiarism. Many educators need help to maintain the integrity of their assignments while students find new ways to leverage AI for shortcuts. In this blog,…
-
In the fast-paced digital world of 2024, mastering the right programming languages can be the key to unlocking exciting career opportunities and innovations. Whether you’re a beginner or an experienced developer, choosing the best languages to learn can make a significant difference in your career trajectory. In this blog, we’ll delve deep into the top programming languages that are set to dominate the…